mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
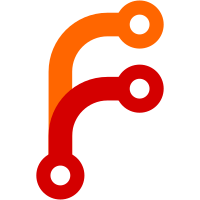
This makes easier to read and debug these tests. This also reduces test lines count by 15% from 3K to 2.5K See https://itnext.io/f-tests-as-a-replacement-for-table-driven-tests-in-go-8814a8b19e9e While at it, consistently use t.Fatal* instead of t.Error*, since t.Error* usually leads to more complicated and fragile tests, while it doesn't bring any practical benefits over t.Fatal*.
36 lines
910 B
Go
36 lines
910 B
Go
package http
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promutils"
|
|
)
|
|
|
|
func TestParseAPIResponse(t *testing.T) {
|
|
f := func(data, path string, resultExpected []httpGroupTarget) {
|
|
t.Helper()
|
|
|
|
result, err := parseAPIResponse([]byte(data), path)
|
|
if err != nil {
|
|
t.Fatalf("parseAPIResponse() error: %s", err)
|
|
}
|
|
if !reflect.DeepEqual(result, resultExpected) {
|
|
t.Fatalf("unexpected result\ngot\n%v\nwant\n%v", result, resultExpected)
|
|
}
|
|
}
|
|
|
|
// parse ok
|
|
data := `[
|
|
{"targets": ["http://target-1:9100","http://target-2:9150"],
|
|
"labels": {"label-1":"value-1"} }
|
|
]`
|
|
path := "/ok"
|
|
resultExpected := []httpGroupTarget{
|
|
{
|
|
Labels: promutils.NewLabelsFromMap(map[string]string{"label-1": "value-1"}),
|
|
Targets: []string{"http://target-1:9100", "http://target-2:9150"},
|
|
},
|
|
}
|
|
f(data, path, resultExpected)
|
|
}
|