mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
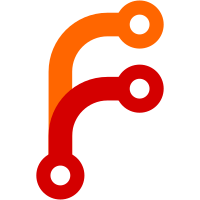
This makes easier to read and debug these tests. This also reduces test lines count by 15% from 3K to 2.5K See https://itnext.io/f-tests-as-a-replacement-for-table-driven-tests-in-go-8814a8b19e9e While at it, consistently use t.Fatal* instead of t.Error*, since t.Error* usually leads to more complicated and fragile tests, while it doesn't bring any practical benefits over t.Fatal*.
163 lines
5.2 KiB
Go
163 lines
5.2 KiB
Go
package yandexcloud
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promscrape/discoveryutils"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promutils"
|
|
)
|
|
|
|
func TestAddInstanceLabels(t *testing.T) {
|
|
f := func(instances []instance, labelssExpected []*promutils.Labels) {
|
|
t.Helper()
|
|
|
|
labelss := addInstanceLabels(instances)
|
|
discoveryutils.TestEqualLabelss(t, labelss, labelssExpected)
|
|
}
|
|
|
|
// empty response
|
|
f(nil, nil)
|
|
|
|
// one server
|
|
instances := []instance{
|
|
{
|
|
Name: "server-1",
|
|
ID: "test",
|
|
FQDN: "server-1.ru-central1.internal",
|
|
FolderID: "test",
|
|
Status: "RUNNING",
|
|
PlatformID: "s2.micro",
|
|
Resources: resources{
|
|
Cores: "2",
|
|
CoreFraction: "20",
|
|
Memory: "4",
|
|
},
|
|
NetworkInterfaces: []networkInterface{
|
|
{
|
|
Index: "0",
|
|
PrimaryV4Address: primaryV4Address{
|
|
Address: "192.168.1.1",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
labelssExpected := []*promutils.Labels{
|
|
promutils.NewLabelsFromMap(map[string]string{
|
|
"__address__": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_name": "server-1",
|
|
"__meta_yandexcloud_instance_fqdn": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_id": "test",
|
|
"__meta_yandexcloud_instance_status": "RUNNING",
|
|
"__meta_yandexcloud_instance_platform_id": "s2.micro",
|
|
"__meta_yandexcloud_instance_resources_cores": "2",
|
|
"__meta_yandexcloud_instance_resources_core_fraction": "20",
|
|
"__meta_yandexcloud_instance_resources_memory": "4",
|
|
"__meta_yandexcloud_folder_id": "test",
|
|
"__meta_yandexcloud_instance_private_ip_0": "192.168.1.1",
|
|
}),
|
|
}
|
|
f(instances, labelssExpected)
|
|
|
|
// with public ip
|
|
instances = []instance{
|
|
{
|
|
Name: "server-1",
|
|
ID: "test",
|
|
FQDN: "server-1.ru-central1.internal",
|
|
FolderID: "test",
|
|
Status: "RUNNING",
|
|
PlatformID: "s2.micro",
|
|
Resources: resources{
|
|
Cores: "2",
|
|
CoreFraction: "20",
|
|
Memory: "4",
|
|
},
|
|
NetworkInterfaces: []networkInterface{
|
|
{
|
|
Index: "0",
|
|
PrimaryV4Address: primaryV4Address{
|
|
Address: "192.168.1.1",
|
|
OneToOneNat: oneToOneNat{
|
|
Address: "1.1.1.1",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
labelssExpected = []*promutils.Labels{
|
|
promutils.NewLabelsFromMap(map[string]string{
|
|
"__address__": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_fqdn": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_name": "server-1",
|
|
"__meta_yandexcloud_instance_id": "test",
|
|
"__meta_yandexcloud_instance_status": "RUNNING",
|
|
"__meta_yandexcloud_instance_platform_id": "s2.micro",
|
|
"__meta_yandexcloud_instance_resources_cores": "2",
|
|
"__meta_yandexcloud_instance_resources_core_fraction": "20",
|
|
"__meta_yandexcloud_instance_resources_memory": "4",
|
|
"__meta_yandexcloud_folder_id": "test",
|
|
"__meta_yandexcloud_instance_private_ip_0": "192.168.1.1",
|
|
"__meta_yandexcloud_instance_public_ip_0": "1.1.1.1",
|
|
}),
|
|
}
|
|
f(instances, labelssExpected)
|
|
|
|
// with dns record
|
|
instances = []instance{
|
|
{
|
|
Name: "server-1",
|
|
ID: "test",
|
|
FQDN: "server-1.ru-central1.internal",
|
|
FolderID: "test",
|
|
Status: "RUNNING",
|
|
PlatformID: "s2.micro",
|
|
Resources: resources{
|
|
Cores: "2",
|
|
CoreFraction: "20",
|
|
Memory: "4",
|
|
},
|
|
NetworkInterfaces: []networkInterface{
|
|
{
|
|
Index: "0",
|
|
PrimaryV4Address: primaryV4Address{
|
|
Address: "192.168.1.1",
|
|
OneToOneNat: oneToOneNat{
|
|
Address: "1.1.1.1",
|
|
DNSRecords: []dnsRecord{
|
|
{
|
|
FQDN: "server-1.example.com",
|
|
},
|
|
},
|
|
},
|
|
DNSRecords: []dnsRecord{
|
|
{
|
|
FQDN: "server-1.example.local",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
labelssExpected = []*promutils.Labels{
|
|
promutils.NewLabelsFromMap(map[string]string{
|
|
"__address__": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_name": "server-1",
|
|
"__meta_yandexcloud_instance_fqdn": "server-1.ru-central1.internal",
|
|
"__meta_yandexcloud_instance_id": "test",
|
|
"__meta_yandexcloud_instance_status": "RUNNING",
|
|
"__meta_yandexcloud_instance_platform_id": "s2.micro",
|
|
"__meta_yandexcloud_instance_resources_cores": "2",
|
|
"__meta_yandexcloud_instance_resources_core_fraction": "20",
|
|
"__meta_yandexcloud_instance_resources_memory": "4",
|
|
"__meta_yandexcloud_folder_id": "test",
|
|
"__meta_yandexcloud_instance_private_ip_0": "192.168.1.1",
|
|
"__meta_yandexcloud_instance_public_ip_0": "1.1.1.1",
|
|
"__meta_yandexcloud_instance_private_dns_0": "server-1.example.local",
|
|
"__meta_yandexcloud_instance_public_dns_0": "server-1.example.com",
|
|
}),
|
|
}
|
|
f(instances, labelssExpected)
|
|
}
|