mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
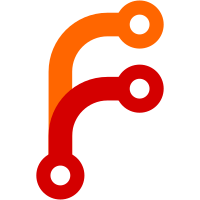
'any' type is supported starting from Go1.18. Let's consistently use it instead of 'interface{}' type across the code base, since `any` is easier to read than 'interface{}'.
62 lines
1.3 KiB
Go
62 lines
1.3 KiB
Go
package discoveryutils
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/VictoriaMetrics/metrics"
|
|
)
|
|
|
|
// ConfigMap is a map for storing discovery api configs.
|
|
//
|
|
// It automatically removes old configs which weren't accessed recently.
|
|
type ConfigMap struct {
|
|
mu sync.Mutex
|
|
m map[any]any
|
|
|
|
entriesCount *metrics.Counter
|
|
}
|
|
|
|
// NewConfigMap creates ConfigMap
|
|
func NewConfigMap() *ConfigMap {
|
|
return &ConfigMap{
|
|
m: make(map[any]any),
|
|
entriesCount: metrics.GetOrCreateCounter(`vm_promscrape_discoveryutils_configmap_entries_count`),
|
|
}
|
|
}
|
|
|
|
// Get returns config for the given key.
|
|
//
|
|
// Key must be a pointer.
|
|
//
|
|
// It creates new config map with newConfig() call if cm doesn't contain config under the given key.
|
|
func (cm *ConfigMap) Get(key any, newConfig func() (any, error)) (any, error) {
|
|
cm.mu.Lock()
|
|
defer cm.mu.Unlock()
|
|
|
|
cfg := cm.m[key]
|
|
if cfg != nil {
|
|
return cfg, nil
|
|
}
|
|
cfg, err := newConfig()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
cm.m[key] = cfg
|
|
cm.entriesCount.Inc()
|
|
return cfg, nil
|
|
}
|
|
|
|
// Delete deletes config for the given key from cm and returns it.
|
|
func (cm *ConfigMap) Delete(key any) any {
|
|
cm.mu.Lock()
|
|
defer cm.mu.Unlock()
|
|
|
|
cfg := cm.m[key]
|
|
if cfg == nil {
|
|
// The cfg can be missing if it wasn't accessed yet.
|
|
return nil
|
|
}
|
|
cm.entriesCount.Dec()
|
|
delete(cm.m, key)
|
|
return cfg
|
|
}
|