mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
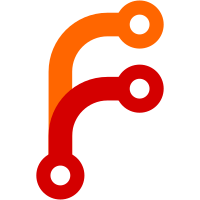
This commit adds the following changes: - Added support to push datadog logs with examples of how to ingest data using Vector and Fluentbit - Updated VictoriaLogs examples directory structure to have single container image for victorialogs, agent (fluentbit, vector, etc) but multiple configurations for different protocols Related issue https://github.com/VictoriaMetrics/VictoriaMetrics/issues/6632
60 lines
1.8 KiB
Go
60 lines
1.8 KiB
Go
package vlinsert
|
|
|
|
import (
|
|
"net/http"
|
|
"strings"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/datadog"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/elasticsearch"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/journald"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/jsonline"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/loki"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/opentelemetry"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/syslog"
|
|
)
|
|
|
|
// Init initializes vlinsert
|
|
func Init() {
|
|
syslog.MustInit()
|
|
}
|
|
|
|
// Stop stops vlinsert
|
|
func Stop() {
|
|
syslog.MustStop()
|
|
}
|
|
|
|
// RequestHandler handles insert requests for VictoriaLogs
|
|
func RequestHandler(w http.ResponseWriter, r *http.Request) bool {
|
|
path := r.URL.Path
|
|
|
|
if !strings.HasPrefix(path, "/insert/") {
|
|
// Skip requests, which do not start with /insert/, since these aren't our requests.
|
|
return false
|
|
}
|
|
path = strings.TrimPrefix(path, "/insert")
|
|
path = strings.ReplaceAll(path, "//", "/")
|
|
|
|
if path == "/jsonline" {
|
|
jsonline.RequestHandler(w, r)
|
|
return true
|
|
}
|
|
switch {
|
|
case strings.HasPrefix(path, "/elasticsearch/"):
|
|
path = strings.TrimPrefix(path, "/elasticsearch")
|
|
return elasticsearch.RequestHandler(path, w, r)
|
|
case strings.HasPrefix(path, "/loki/"):
|
|
path = strings.TrimPrefix(path, "/loki")
|
|
return loki.RequestHandler(path, w, r)
|
|
case strings.HasPrefix(path, "/opentelemetry/"):
|
|
path = strings.TrimPrefix(path, "/opentelemetry")
|
|
return opentelemetry.RequestHandler(path, w, r)
|
|
case strings.HasPrefix(path, "/journald/"):
|
|
path = strings.TrimPrefix(path, "/journald")
|
|
return journald.RequestHandler(path, w, r)
|
|
case strings.HasPrefix(path, "/datadog/"):
|
|
path = strings.TrimPrefix(path, "/datadog")
|
|
return datadog.RequestHandler(path, w, r)
|
|
default:
|
|
return false
|
|
}
|
|
}
|