mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2025-02-09 15:27:11 +00:00
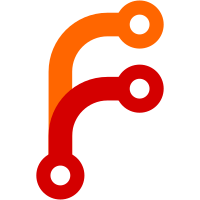
Use chunked allocator in order to reduce memory allocations. It allocates objects from slices of up to 64Kb size. This improves performance for `stats` and `top` pipes by up to 2x when they are applied to big number of `by (...)` groups. Also parallelize execution of `count_uniq`, `count_uniq_hash` and `uniq_values` stats functions, so they are executed faster on hosts with many CPU cores when applied to fields with big number of unique values.
364 lines
4.3 KiB
Go
364 lines
4.3 KiB
Go
package logstorage
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func TestParseStatsMedianSuccess(t *testing.T) {
|
|
f := func(pipeStr string) {
|
|
t.Helper()
|
|
expectParseStatsFuncSuccess(t, pipeStr)
|
|
}
|
|
|
|
f(`median(*)`)
|
|
f(`median(a)`)
|
|
f(`median(a, b)`)
|
|
}
|
|
|
|
func TestParseStatsMedianFailure(t *testing.T) {
|
|
f := func(pipeStr string) {
|
|
t.Helper()
|
|
expectParseStatsFuncFailure(t, pipeStr)
|
|
}
|
|
|
|
f(`median`)
|
|
f(`median(a b)`)
|
|
f(`median(x) y`)
|
|
}
|
|
|
|
func TestStatsMedian(t *testing.T) {
|
|
f := func(pipeStr string, rows, rowsExpected [][]Field) {
|
|
t.Helper()
|
|
expectPipeResults(t, pipeStr, rows, rowsExpected)
|
|
}
|
|
|
|
f("stats median(*) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats median(a) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", "2"},
|
|
},
|
|
})
|
|
|
|
f("stats median(a, b) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats median(b) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats median(c) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", ""},
|
|
},
|
|
})
|
|
|
|
f("stats median(a) if (b:*) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats by (b) median(a) if (b:*) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `2`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
{"b", "3"},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"c", `54`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"b", "3"},
|
|
{"x", "2"},
|
|
},
|
|
{
|
|
{"b", ""},
|
|
{"x", ""},
|
|
},
|
|
})
|
|
|
|
f("stats by (a) median(b) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `5`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `7`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"x", "3"},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"x", "7"},
|
|
},
|
|
})
|
|
|
|
f("stats by (a) median(*) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
{"c", "3"},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `5`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `7`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"x", "3"},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"x", "5"},
|
|
},
|
|
})
|
|
|
|
f("stats by (a) median(c) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"c", `5`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `7`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"x", ""},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"x", "5"},
|
|
},
|
|
})
|
|
|
|
f("stats by (a) median(a, b, c) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
{"c", "3"},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `5`},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `7`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"x", "1"},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats by (a, b) median(a) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
{"c", "3"},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `5`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"b", "3"},
|
|
{"x", "1"},
|
|
},
|
|
{
|
|
{"a", "1"},
|
|
{"b", ""},
|
|
{"x", "1"},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"b", "5"},
|
|
{"x", "3"},
|
|
},
|
|
})
|
|
|
|
f("stats by (a, b) median(c) as x", [][]Field{
|
|
{
|
|
{"_msg", `abc`},
|
|
{"a", `1`},
|
|
{"b", `3`},
|
|
},
|
|
{
|
|
{"_msg", `def`},
|
|
{"a", `1`},
|
|
{"c", "3"},
|
|
},
|
|
{
|
|
{"a", `3`},
|
|
{"b", `5`},
|
|
},
|
|
}, [][]Field{
|
|
{
|
|
{"a", "1"},
|
|
{"b", "3"},
|
|
{"x", ""},
|
|
},
|
|
{
|
|
{"a", "1"},
|
|
{"b", ""},
|
|
{"x", "3"},
|
|
},
|
|
{
|
|
{"a", "3"},
|
|
{"b", "5"},
|
|
{"x", ""},
|
|
},
|
|
})
|
|
}
|