mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
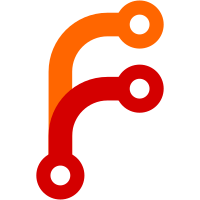
Consistently using t.Fatal* simplifies the test code and makes it less fragile, since it is common error
to forget to make proper cleanup after t.Error* call. Also t.Error* calls do not provide any practical
benefits when some tests fail. They just clutter test output with additional noise information,
which do not help in fixing failing tests most of the time.
This is a follow-up for a9525da8a4
37 lines
1 KiB
Go
37 lines
1 KiB
Go
package main
|
|
|
|
import (
|
|
"path/filepath"
|
|
"testing"
|
|
)
|
|
|
|
func TestHasFilepathPrefix(t *testing.T) {
|
|
f := func(dst, storageDataPath string, resultExpected bool) {
|
|
t.Helper()
|
|
|
|
result := hasFilepathPrefix(dst, storageDataPath)
|
|
if result != resultExpected {
|
|
t.Fatalf("unexpected hasFilepathPrefix(%q, %q); got: %v; want: %v", dst, storageDataPath, result, resultExpected)
|
|
}
|
|
}
|
|
|
|
pwd, err := filepath.Abs("")
|
|
if err != nil {
|
|
t.Fatalf("cannot determine working directory: %s", err)
|
|
}
|
|
|
|
f("s3://foo/bar", "foo", false)
|
|
f("fs://"+pwd+"/foo", "foo", true)
|
|
f("fs://"+pwd+"/foo", "foo/bar", false)
|
|
f("fs://"+pwd+"/foo/bar", "foo", true)
|
|
f("fs://"+pwd+"/foo", "bar", false)
|
|
f("fs://"+pwd+"/foo", pwd+"/foo", true)
|
|
f("fs://"+pwd+"/foo", pwd+"/foo/bar", false)
|
|
f("fs://"+pwd+"/foo/bar", pwd+"/foo", true)
|
|
f("fs://"+pwd+"/foo", pwd+"/bar", false)
|
|
f("fs:///data1", "/data", false)
|
|
f("fs:///data", "/data1", false)
|
|
f("fs:///data", "/data/foo", false)
|
|
f("fs:///data/foo", "/data", true)
|
|
f("fs:///data/foo/", "/data/", true)
|
|
}
|