mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
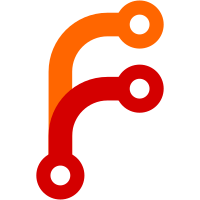
### Describe Your Changes Added an ability to query data across multiple tenants. See: https://github.com/VictoriaMetrics/VictoriaMetrics/issues/1434 Currently, the following endpoints work with multi-tenancy: - /prometheus/api/v1/query - /prometheus/api/v1/query_range - /prometheus/api/v1/series - /prometheus/api/v1/labels - /prometheus/api/v1/label/<label_name>/values - /prometheus/api/v1/status/active_queries - /prometheus/api/v1/status/top_queries - /prometheus/api/v1/status/tsdb - /prometheus/api/v1/export - /prometheus/api/v1/export/csv - /vmui A note regarding VMUI: endpoints such as `active_queries` and `top_queries` have been updated to indicate whether query was a single-tenant or multi-tenant, but UI needs to be updated to display this info. cc: @Loori-R --------- Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> Signed-off-by: f41gh7 <nik@victoriametrics.com> Co-authored-by: f41gh7 <nik@victoriametrics.com>
82 lines
2 KiB
Go
82 lines
2 KiB
Go
package auth
|
|
|
|
import (
|
|
"fmt"
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
// Token contains settings for request processing
|
|
type Token struct {
|
|
AccountID uint32
|
|
ProjectID uint32
|
|
}
|
|
|
|
// String returns string representation of t.
|
|
func (t *Token) String() string {
|
|
if t == nil {
|
|
return "multitenant"
|
|
}
|
|
if t.ProjectID == 0 {
|
|
return fmt.Sprintf("%d", t.AccountID)
|
|
}
|
|
return fmt.Sprintf("%d:%d", t.AccountID, t.ProjectID)
|
|
}
|
|
|
|
// NewToken returns new Token for the given authToken.
|
|
func NewToken(authToken string) (*Token, error) {
|
|
var t Token
|
|
if err := t.Init(authToken); err != nil {
|
|
return nil, err
|
|
}
|
|
return &t, nil
|
|
}
|
|
|
|
// NewTokenPossibleMultitenant returns new Token for the given authToken.
|
|
//
|
|
// If authToken == "multitenant", then nil Token is returned.
|
|
func NewTokenPossibleMultitenant(authToken string) (*Token, error) {
|
|
if authToken == "multitenant" {
|
|
return nil, nil
|
|
}
|
|
return NewToken(authToken)
|
|
}
|
|
|
|
// Init initializes t from authToken.
|
|
func (t *Token) Init(authToken string) error {
|
|
accountID, projectID, err := ParseToken(authToken)
|
|
if err != nil {
|
|
return fmt.Errorf("cannot parse authToken %q: %w", authToken, err)
|
|
}
|
|
|
|
t.Set(accountID, projectID)
|
|
return nil
|
|
}
|
|
|
|
// ParseToken parses authToken and returns accountID and projectID from it.
|
|
func ParseToken(authToken string) (uint32, uint32, error) {
|
|
tmp := strings.Split(authToken, ":")
|
|
if len(tmp) > 2 {
|
|
return 0, 0, fmt.Errorf("unexpected number of items in authToken %q; got %d; want 1 or 2", authToken, len(tmp))
|
|
}
|
|
n, err := strconv.ParseUint(tmp[0], 10, 32)
|
|
if err != nil {
|
|
return 0, 0, fmt.Errorf("cannot parse accountID from %q: %w", tmp[0], err)
|
|
}
|
|
accountID := uint32(n)
|
|
projectID := uint32(0)
|
|
if len(tmp) > 1 {
|
|
n, err := strconv.ParseUint(tmp[1], 10, 32)
|
|
if err != nil {
|
|
return 0, 0, fmt.Errorf("cannot parse projectID from %q: %w", tmp[1], err)
|
|
}
|
|
projectID = uint32(n)
|
|
}
|
|
return accountID, projectID, nil
|
|
}
|
|
|
|
// Set sets accountID and projectID for the t.
|
|
func (t *Token) Set(accountID, projectID uint32) {
|
|
t.AccountID = accountID
|
|
t.ProjectID = projectID
|
|
}
|