mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
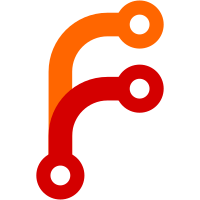
### Describe Your Changes If a dict flag has only one value without a prefix it is supposed to replace default value. Previously, when flag was set to `-flag=2` and the default value in `NewDictInt` was set to 1 the resulting value for any `flag.Get()` call would be 1 which is not expected. This commit updates default value for the flag in case there is only one entry for flag and the entry is a number without a key. This affects cluster version and specifically `replicationFactor` flag usage with vmstorage [node groups](https://docs.victoriametrics.com/cluster-victoriametrics/#vmstorage-groups-at-vmselect). Previously, the following configuration would effectively be ignored: ``` /path/to/vmselect \ -replicationFactor=2 \ -storageNode=g1/host1,g1/host2,g1/host3 \ -storageNode=g2/host4,g2/host5,g2/host6 \ -storageNode=g3/host7,g3/host8,g3/host9 ``` Changes from this PR will force default value for `replicationFactor` flag to be set to `2` which is expected as the result of this configuration. --------- Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com>
113 lines
2 KiB
Go
113 lines
2 KiB
Go
package flagutil
|
|
|
|
import (
|
|
"encoding/json"
|
|
"testing"
|
|
)
|
|
|
|
func TestParseJSONMapSuccess(t *testing.T) {
|
|
f := func(s string) {
|
|
t.Helper()
|
|
m, err := ParseJSONMap(s)
|
|
if err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
if s == "" && m == nil {
|
|
return
|
|
}
|
|
data, err := json.Marshal(m)
|
|
if err != nil {
|
|
t.Fatalf("cannot marshal m: %s", err)
|
|
}
|
|
if s != string(data) {
|
|
t.Fatalf("unexpected result; got %s; want %s", data, s)
|
|
}
|
|
}
|
|
|
|
f("")
|
|
f("{}")
|
|
f(`{"foo":"bar"}`)
|
|
f(`{"a":"b","c":"d"}`)
|
|
}
|
|
|
|
func TestParseJSONMapFailure(t *testing.T) {
|
|
f := func(s string) {
|
|
t.Helper()
|
|
m, err := ParseJSONMap(s)
|
|
if err == nil {
|
|
t.Fatalf("expecting non-nil error")
|
|
}
|
|
if m != nil {
|
|
t.Fatalf("expecting nil m")
|
|
}
|
|
}
|
|
|
|
f("foo")
|
|
f("123")
|
|
f("{")
|
|
f(`{foo:bar}`)
|
|
f(`{"foo":1}`)
|
|
f(`[]`)
|
|
f(`{"foo":"bar","a":[123]}`)
|
|
}
|
|
|
|
func TestDictIntSetSuccess(t *testing.T) {
|
|
f := func(s string) {
|
|
t.Helper()
|
|
var di DictInt
|
|
if err := di.Set(s); err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
result := di.String()
|
|
if result != s {
|
|
t.Fatalf("unexpected DictInt.String(); got %q; want %q", result, s)
|
|
}
|
|
}
|
|
|
|
f("123")
|
|
f("-234")
|
|
f("foo:123")
|
|
f("foo:123,bar:-42,baz:0,aa:43")
|
|
}
|
|
|
|
func TestDictIntFailure(t *testing.T) {
|
|
f := func(s string) {
|
|
t.Helper()
|
|
var di DictInt
|
|
if err := di.Set(s); err == nil {
|
|
t.Fatalf("expecting non-nil error")
|
|
}
|
|
}
|
|
|
|
// missing values
|
|
f("foo")
|
|
f("foo:")
|
|
|
|
// non-integer values
|
|
f("foo:bar")
|
|
f("12.34")
|
|
f("foo:123.34")
|
|
|
|
// duplicate keys
|
|
f("a:234,k:123,k:432")
|
|
}
|
|
|
|
func TestDictIntGet(t *testing.T) {
|
|
f := func(s, key string, defaultValue, expectedValue int) {
|
|
t.Helper()
|
|
var di DictInt
|
|
di.defaultValue = defaultValue
|
|
if err := di.Set(s); err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
value := di.Get(key)
|
|
if value != expectedValue {
|
|
t.Fatalf("unexpected value; got %d; want %d", value, expectedValue)
|
|
}
|
|
}
|
|
|
|
f("foo:42", "", 123, 123)
|
|
f("foo:42", "foo", 123, 42)
|
|
f("532", "", 123, 532)
|
|
f("532", "foo", 123, 532)
|
|
}
|