mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
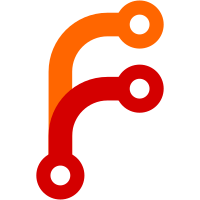
### Describe Your Changes Added an ability to query data across multiple tenants. See: https://github.com/VictoriaMetrics/VictoriaMetrics/issues/1434 Currently, the following endpoints work with multi-tenancy: - /prometheus/api/v1/query - /prometheus/api/v1/query_range - /prometheus/api/v1/series - /prometheus/api/v1/labels - /prometheus/api/v1/label/<label_name>/values - /prometheus/api/v1/status/active_queries - /prometheus/api/v1/status/top_queries - /prometheus/api/v1/status/tsdb - /prometheus/api/v1/export - /prometheus/api/v1/export/csv - /vmui A note regarding VMUI: endpoints such as `active_queries` and `top_queries` have been updated to indicate whether query was a single-tenant or multi-tenant, but UI needs to be updated to display this info. cc: @Loori-R --------- Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> Signed-off-by: f41gh7 <nik@victoriametrics.com> Co-authored-by: f41gh7 <nik@victoriametrics.com>
31 lines
764 B
Go
31 lines
764 B
Go
package timeutil
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/valyala/fastrand"
|
|
)
|
|
|
|
// AddJitterToDuration adds up to 10% random jitter to d and returns the resulting duration.
|
|
//
|
|
// The maximum jitter is limited by 10 seconds.
|
|
func AddJitterToDuration(d time.Duration) time.Duration {
|
|
dv := d / 10
|
|
if dv > 10*time.Second {
|
|
dv = 10 * time.Second
|
|
}
|
|
p := float64(fastrand.Uint32()) / (1 << 32)
|
|
return d + time.Duration(p*float64(dv))
|
|
}
|
|
|
|
// StartOfDay returns the start of the day for the given timestamp.
|
|
// Timestamp is in milliseconds.
|
|
func StartOfDay(ts int64) int64 {
|
|
return ts - (ts % 86400000)
|
|
}
|
|
|
|
// EndOfDay returns the end of the day for the given timestamp.
|
|
// Timestamp is in milliseconds.
|
|
func EndOfDay(ts int64) int64 {
|
|
return StartOfDay(ts) + 86400000 - 1
|
|
}
|