mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
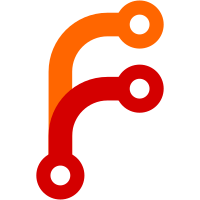
* vmalert: introduce additional HTTP URL params per-group configuration The new group field `params` allows to configure custom HTTP URL params per each group. These params will be applied to every request before executing rule's expression. Hot config reload is also supported. Field `extra_filter_labels` was deprecated in favour of `params` field. vmalert will print deprecation log message if config file contains the deprecated field. `params` fields are supported by both Prometheus and Graphite datasource types. Signed-off-by: hagen1778 <roman@victoriametrics.com> * vmalert: provide more examples for `params` field Signed-off-by: hagen1778 <roman@victoriametrics.com> * vmalert: set higher priority for `params` setting If there would be a conflict between URL params set in `datasource.url` flag and params in group definition the latter will have higher priority. Signed-off-by: hagen1778 <roman@victoriametrics.com>
66 lines
1.4 KiB
Go
66 lines
1.4 KiB
Go
package datasource
|
|
|
|
import (
|
|
"context"
|
|
"net/url"
|
|
"time"
|
|
)
|
|
|
|
// Querier interface wraps Query and QueryRange methods
|
|
type Querier interface {
|
|
Query(ctx context.Context, query string) ([]Metric, error)
|
|
QueryRange(ctx context.Context, query string, from, to time.Time) ([]Metric, error)
|
|
}
|
|
|
|
// QuerierBuilder builds Querier with given params.
|
|
type QuerierBuilder interface {
|
|
BuildWithParams(params QuerierParams) Querier
|
|
}
|
|
|
|
// QuerierParams params for Querier.
|
|
type QuerierParams struct {
|
|
DataSourceType *Type
|
|
EvaluationInterval time.Duration
|
|
QueryParams url.Values
|
|
}
|
|
|
|
// Metric is the basic entity which should be return by datasource
|
|
type Metric struct {
|
|
Labels []Label
|
|
Timestamps []int64
|
|
Values []float64
|
|
}
|
|
|
|
// SetLabel adds or updates existing one label
|
|
// by the given key and label
|
|
func (m *Metric) SetLabel(key, value string) {
|
|
for i, l := range m.Labels {
|
|
if l.Name == key {
|
|
m.Labels[i].Value = value
|
|
return
|
|
}
|
|
}
|
|
m.AddLabel(key, value)
|
|
}
|
|
|
|
// AddLabel appends the given label to the label set
|
|
func (m *Metric) AddLabel(key, value string) {
|
|
m.Labels = append(m.Labels, Label{Name: key, Value: value})
|
|
}
|
|
|
|
// Label returns the given label value.
|
|
// If label is missing empty string will be returned
|
|
func (m *Metric) Label(key string) string {
|
|
for _, l := range m.Labels {
|
|
if l.Name == key {
|
|
return l.Value
|
|
}
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Label represents metric's label
|
|
type Label struct {
|
|
Name string
|
|
Value string
|
|
}
|