mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
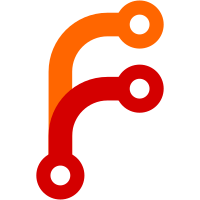
* app/vlinsert: add support of loki push protocol - implemented loki push protocol for both Protobuf and JSON formats - added examples in documentation - added example docker-compose Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * app/vlinsert: move protobuf metric into its own file Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * deployment/docker/victorialogs/promtail: update reference to docker image Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * deployment/docker/victorialogs/promtail: make volume name unique Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * app/vlinsert/loki: add license reference Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * deployment/docker/victorialogs/promtail: fix volume name Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * docs/VictoriaLogs/data-ingestion: add stream fields for loki JSON ingestion example Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * app/vlinsert/loki: move entities to places where those are used Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * app/vlinsert/loki: refactor to use common components - use CommonParameters from insertutils - stop ingestion after first error similar to elasticsearch and jsonline Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> * app/vlinsert/loki: address review feedback - add missing logstorage.PutLogRows calls - refactor tenant ID parsing to use common function - reduce number of allocations for parsing by reusing logfields slices - add tests and benchmarks for requests processing funcs Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com> --------- Signed-off-by: Zakhar Bessarab <z.bessarab@victoriametrics.com>
59 lines
1.5 KiB
Go
59 lines
1.5 KiB
Go
package loki
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vlinsert/insertutils"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/logger"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/logstorage"
|
|
"github.com/VictoriaMetrics/metrics"
|
|
)
|
|
|
|
const msgField = "_msg"
|
|
|
|
var (
|
|
lokiRequestsTotal = metrics.NewCounter(`vl_http_requests_total{path="/insert/loki/api/v1/push"}`)
|
|
)
|
|
|
|
// RequestHandler processes ElasticSearch insert requests
|
|
func RequestHandler(path string, w http.ResponseWriter, r *http.Request) bool {
|
|
switch path {
|
|
case "/api/v1/push":
|
|
contentType := r.Header.Get("Content-Type")
|
|
lokiRequestsTotal.Inc()
|
|
switch contentType {
|
|
case "application/x-protobuf":
|
|
return handleProtobuf(r, w)
|
|
case "application/json", "gzip":
|
|
return handleJSON(r, w)
|
|
default:
|
|
logger.Warnf("unsupported Content-Type=%q for %q request; skipping it", contentType, path)
|
|
return false
|
|
}
|
|
default:
|
|
return false
|
|
}
|
|
}
|
|
|
|
func getCommonParams(r *http.Request) (*insertutils.CommonParams, error) {
|
|
cp, err := insertutils.GetCommonParams(r)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// If parsed tenant is (0,0) it is likely to be default tenant
|
|
// Try parsing tenant from Loki headers
|
|
if cp.TenantID.AccountID == 0 && cp.TenantID.ProjectID == 0 {
|
|
org := r.Header.Get("X-Scope-OrgID")
|
|
if org != "" {
|
|
tenantID, err := logstorage.GetTenantIDFromString(org)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
cp.TenantID = tenantID
|
|
}
|
|
|
|
}
|
|
|
|
return cp, nil
|
|
}
|