mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
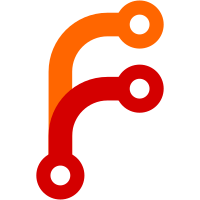
There are real-world cases when TCP connection needs more than 1 second to be established.
69 lines
1.5 KiB
Go
69 lines
1.5 KiB
Go
package netutil
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"time"
|
|
|
|
"github.com/VictoriaMetrics/metrics"
|
|
)
|
|
|
|
// NewTCPDialer returns new dialer for dialing the given addr.
|
|
//
|
|
// The name is used in metric tags for the returned dialer.
|
|
// The name must be unique among dialers.
|
|
func NewTCPDialer(name, addr string) *TCPDialer {
|
|
d := &TCPDialer{
|
|
d: &net.Dialer{
|
|
// The timeout for establishing a TCP connection.
|
|
// 5 seconds should be enough for the majority of cases.
|
|
Timeout: 5 * time.Second,
|
|
|
|
// How frequently to send keep-alive packets over established TCP connections.
|
|
KeepAlive: time.Second,
|
|
},
|
|
|
|
addr: addr,
|
|
|
|
dials: metrics.NewCounter(fmt.Sprintf(`vm_tcpdialer_dials_total{name=%q, addr=%q}`, name, addr)),
|
|
dialErrors: metrics.NewCounter(fmt.Sprintf(`vm_tcpdialer_errors_total{name=%q, addr=%q, type="dial"}`, name, addr)),
|
|
}
|
|
d.connMetrics.init("vm_tcpdialer", name, addr)
|
|
return d
|
|
}
|
|
|
|
// TCPDialer is used for dialing the addr passed to NewTCPDialer.
|
|
//
|
|
// It also gathers various stats for dialed connections.
|
|
type TCPDialer struct {
|
|
d *net.Dialer
|
|
|
|
addr string
|
|
|
|
dials *metrics.Counter
|
|
dialErrors *metrics.Counter
|
|
|
|
connMetrics
|
|
}
|
|
|
|
// Dial dials the addr passed to NewTCPDialer.
|
|
func (d *TCPDialer) Dial() (net.Conn, error) {
|
|
d.dials.Inc()
|
|
network := GetTCPNetwork()
|
|
c, err := d.d.Dial(network, d.addr)
|
|
if err != nil {
|
|
d.dialErrors.Inc()
|
|
return nil, err
|
|
}
|
|
d.conns.Inc()
|
|
sc := &statConn{
|
|
Conn: c,
|
|
cm: &d.connMetrics,
|
|
}
|
|
return sc, err
|
|
}
|
|
|
|
// Addr returns the address the dialer dials to.
|
|
func (d *TCPDialer) Addr() string {
|
|
return d.addr
|
|
}
|