mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
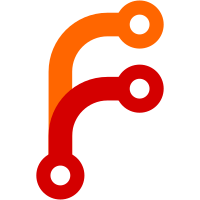
This can be done via extra_fields query arg or via VL-Extra-Fields HTTP header. See https://github.com/VictoriaMetrics/VictoriaMetrics/issues/7354#issuecomment-2448671445
145 lines
3.4 KiB
Go
145 lines
3.4 KiB
Go
package logstorage
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestLogRows_DefaultMsgValue(t *testing.T) {
|
|
type opts struct {
|
|
rows []string
|
|
|
|
streamFields []string
|
|
ignoreFields []string
|
|
extraFields []Field
|
|
defaultMsgValue string
|
|
|
|
resultExpected []string
|
|
}
|
|
|
|
f := func(o opts) {
|
|
t.Helper()
|
|
|
|
lr := GetLogRows(o.streamFields, o.ignoreFields, o.extraFields, o.defaultMsgValue)
|
|
defer PutLogRows(lr)
|
|
|
|
tid := TenantID{
|
|
AccountID: 123,
|
|
ProjectID: 456,
|
|
}
|
|
|
|
p := GetJSONParser()
|
|
defer PutJSONParser(p)
|
|
for i, r := range o.rows {
|
|
if err := p.ParseLogMessage([]byte(r)); err != nil {
|
|
t.Fatalf("unexpected error when parsing %q: %s", r, err)
|
|
}
|
|
timestamp := int64(i)*1_000 + 1
|
|
lr.MustAdd(tid, timestamp, p.Fields)
|
|
}
|
|
|
|
var result []string
|
|
for i := range o.rows {
|
|
s := lr.GetRowString(i)
|
|
result = append(result, s)
|
|
}
|
|
if !reflect.DeepEqual(result, o.resultExpected) {
|
|
t.Fatalf("unexpected result\ngot\n%v\nwant\n%v", result, o.resultExpected)
|
|
}
|
|
}
|
|
|
|
var o opts
|
|
|
|
f(o)
|
|
|
|
// default options
|
|
o = opts{
|
|
rows: []string{
|
|
`{"foo":"bar"}`,
|
|
`{}`,
|
|
`{"foo":"bar","a":"b"}`,
|
|
},
|
|
resultExpected: []string{
|
|
`{"_stream":"{}","_time":"1970-01-01T00:00:00.000000001Z","foo":"bar"}`,
|
|
`{"_stream":"{}","_time":"1970-01-01T00:00:00.000001001Z"}`,
|
|
`{"_stream":"{}","_time":"1970-01-01T00:00:00.000002001Z","a":"b","foo":"bar"}`,
|
|
},
|
|
}
|
|
f(o)
|
|
|
|
// stream fields
|
|
o = opts{
|
|
rows: []string{
|
|
`{"x":"y","foo":"bar"}`,
|
|
`{"x":"y","foo":"bar","abc":"de"}`,
|
|
`{}`,
|
|
},
|
|
streamFields: []string{"foo", "abc"},
|
|
resultExpected: []string{
|
|
`{"_stream":"{foo=\"bar\"}","_time":"1970-01-01T00:00:00.000000001Z","foo":"bar","x":"y"}`,
|
|
`{"_stream":"{abc=\"de\",foo=\"bar\"}","_time":"1970-01-01T00:00:00.000001001Z","abc":"de","foo":"bar","x":"y"}`,
|
|
`{"_stream":"{}","_time":"1970-01-01T00:00:00.000002001Z"}`,
|
|
},
|
|
}
|
|
f(o)
|
|
|
|
// ignore fields
|
|
o = opts{
|
|
rows: []string{
|
|
`{"x":"y","foo":"bar"}`,
|
|
`{"x":"y"}`,
|
|
`{}`,
|
|
},
|
|
streamFields: []string{"foo", "abc", "x"},
|
|
ignoreFields: []string{"foo"},
|
|
resultExpected: []string{
|
|
`{"_stream":"{x=\"y\"}","_time":"1970-01-01T00:00:00.000000001Z","x":"y"}`,
|
|
`{"_stream":"{x=\"y\"}","_time":"1970-01-01T00:00:00.000001001Z","x":"y"}`,
|
|
`{"_stream":"{}","_time":"1970-01-01T00:00:00.000002001Z"}`,
|
|
},
|
|
}
|
|
f(o)
|
|
|
|
// extra fields
|
|
o = opts{
|
|
rows: []string{
|
|
`{"x":"y","foo":"bar"}`,
|
|
`{}`,
|
|
},
|
|
streamFields: []string{"foo", "abc", "x"},
|
|
ignoreFields: []string{"foo"},
|
|
extraFields: []Field{
|
|
{
|
|
Name: "foo",
|
|
Value: "test",
|
|
},
|
|
{
|
|
Name: "abc",
|
|
Value: "1234",
|
|
},
|
|
},
|
|
resultExpected: []string{
|
|
`{"_stream":"{abc=\"1234\",foo=\"test\",x=\"y\"}","_time":"1970-01-01T00:00:00.000000001Z","abc":"1234","foo":"test","x":"y"}`,
|
|
`{"_stream":"{abc=\"1234\",foo=\"test\"}","_time":"1970-01-01T00:00:00.000001001Z","abc":"1234","foo":"test"}`,
|
|
},
|
|
}
|
|
f(o)
|
|
|
|
// default _msg value
|
|
o = opts{
|
|
rows: []string{
|
|
`{"x":"y","foo":"bar"}`,
|
|
`{"_msg":"ppp"}`,
|
|
`{"abc":"ppp"}`,
|
|
},
|
|
streamFields: []string{"abc", "x"},
|
|
defaultMsgValue: "qwert",
|
|
resultExpected: []string{
|
|
`{"_msg":"qwert","_stream":"{x=\"y\"}","_time":"1970-01-01T00:00:00.000000001Z","foo":"bar","x":"y"}`,
|
|
`{"_msg":"ppp","_stream":"{}","_time":"1970-01-01T00:00:00.000001001Z"}`,
|
|
`{"_msg":"qwert","_stream":"{abc=\"ppp\"}","_time":"1970-01-01T00:00:00.000002001Z","abc":"ppp"}`,
|
|
},
|
|
}
|
|
f(o)
|
|
|
|
}
|