mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
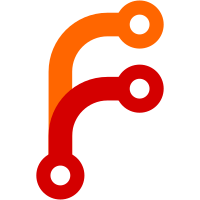
Cache sanitized label names and return them next time. This reduces the number of allocations and speeds up the SanitizeLabelName() function for common case when the number of unique label names is smaller than 100k
56 lines
1.1 KiB
Go
56 lines
1.1 KiB
Go
package discoveryutils
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestSanitizeLabelNameSerial(t *testing.T) {
|
|
if err := testSanitizeLabelName(); err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
}
|
|
|
|
func TestSanitizeLabelNameParallel(t *testing.T) {
|
|
goroutines := 5
|
|
ch := make(chan error, goroutines)
|
|
for i := 0; i < goroutines; i++ {
|
|
go func() {
|
|
ch <- testSanitizeLabelName()
|
|
}()
|
|
}
|
|
tch := time.After(5 * time.Second)
|
|
for i := 0; i < goroutines; i++ {
|
|
select {
|
|
case <-tch:
|
|
t.Fatalf("timeout!")
|
|
case err := <-ch:
|
|
if err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
func testSanitizeLabelName() error {
|
|
f := func(name, expectedSanitizedName string) error {
|
|
for i := 0; i < 5; i++ {
|
|
sanitizedName := SanitizeLabelName(name)
|
|
if sanitizedName != expectedSanitizedName {
|
|
return fmt.Errorf("unexpected sanitized label name %q; got %q; want %q", name, sanitizedName, expectedSanitizedName)
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
if err := f("", ""); err != nil {
|
|
return err
|
|
}
|
|
if err := f("foo", "foo"); err != nil {
|
|
return err
|
|
}
|
|
if err := f("foo-bar/baz", "foo_bar_baz"); err != nil {
|
|
return err
|
|
}
|
|
return nil
|
|
}
|