mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
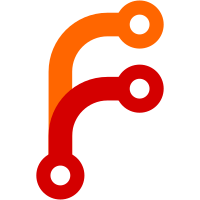
This allows copy-n-pasting the url to another browser window and seeing the same result. The limit in 1000 chars is selected in order to prevent from potential issues with systems which limit the url length such as Internet Explorer - see https://stackoverflow.com/questions/812925/what-is-the-maximum-possible-length-of-a-query-string If the limit is exceeded, then query args are sent via POST method and aren't visible in the url. Updates https://github.com/VictoriaMetrics/VictoriaMetrics/issues/3580
48 lines
1.4 KiB
Go
48 lines
1.4 KiB
Go
package promscrape
|
|
|
|
import (
|
|
"fmt"
|
|
"net/http"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promrelabel"
|
|
)
|
|
|
|
// WriteMetricRelabelDebug serves requests to /metric-relabel-debug page
|
|
func WriteMetricRelabelDebug(w http.ResponseWriter, r *http.Request) {
|
|
targetID := r.FormValue("id")
|
|
metric := r.FormValue("metric")
|
|
relabelConfigs := r.FormValue("relabel_configs")
|
|
var err error
|
|
|
|
if metric == "" && relabelConfigs == "" && targetID != "" {
|
|
pcs, labels, ok := getMetricRelabelContextByTargetID(targetID)
|
|
if !ok {
|
|
err = fmt.Errorf("cannot find target for id=%s", targetID)
|
|
targetID = ""
|
|
} else {
|
|
metric = labels.String()
|
|
relabelConfigs = pcs.String()
|
|
}
|
|
}
|
|
promrelabel.WriteMetricRelabelDebug(w, targetID, metric, relabelConfigs, err)
|
|
}
|
|
|
|
// WriteTargetRelabelDebug generates response for /target-relabel-debug page
|
|
func WriteTargetRelabelDebug(w http.ResponseWriter, r *http.Request) {
|
|
targetID := r.FormValue("id")
|
|
metric := r.FormValue("metric")
|
|
relabelConfigs := r.FormValue("relabel_configs")
|
|
var err error
|
|
|
|
if metric == "" && relabelConfigs == "" && targetID != "" {
|
|
pcs, labels, ok := getTargetRelabelContextByTargetID(targetID)
|
|
if !ok {
|
|
err = fmt.Errorf("cannot find target for id=%s", targetID)
|
|
targetID = ""
|
|
} else {
|
|
metric = labels.String()
|
|
relabelConfigs = pcs.String()
|
|
}
|
|
}
|
|
promrelabel.WriteTargetRelabelDebug(w, targetID, metric, relabelConfigs, err)
|
|
}
|