mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
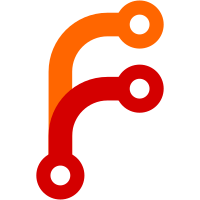
This should reduce the time needed for aggregation state flush on systems with many CPU cores
153 lines
3.4 KiB
Go
153 lines
3.4 KiB
Go
package streamaggr
|
|
|
|
import (
|
|
"math"
|
|
"strings"
|
|
"sync"
|
|
"time"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/fasttime"
|
|
)
|
|
|
|
// totalAggrState calculates output=total, e.g. the summary counter over input counters.
|
|
type totalAggrState struct {
|
|
m sync.Map
|
|
|
|
suffix string
|
|
resetTotalOnFlush bool
|
|
keepFirstSample bool
|
|
stalenessSecs uint64
|
|
}
|
|
|
|
type totalStateValue struct {
|
|
mu sync.Mutex
|
|
lastValues map[string]*lastValueState
|
|
total float64
|
|
deleteDeadline uint64
|
|
deleted bool
|
|
}
|
|
|
|
type lastValueState struct {
|
|
value float64
|
|
deleteDeadline uint64
|
|
}
|
|
|
|
func newTotalAggrState(stalenessInterval time.Duration, resetTotalOnFlush, keepFirstSample bool) *totalAggrState {
|
|
stalenessSecs := roundDurationToSecs(stalenessInterval)
|
|
suffix := "total"
|
|
if resetTotalOnFlush {
|
|
suffix = "increase"
|
|
}
|
|
return &totalAggrState{
|
|
suffix: suffix,
|
|
resetTotalOnFlush: resetTotalOnFlush,
|
|
keepFirstSample: keepFirstSample,
|
|
stalenessSecs: stalenessSecs,
|
|
}
|
|
}
|
|
|
|
func (as *totalAggrState) pushSamples(samples []pushSample) {
|
|
deleteDeadline := fasttime.UnixTimestamp() + as.stalenessSecs
|
|
for i := range samples {
|
|
s := &samples[i]
|
|
inputKey, outputKey := getInputOutputKey(s.key)
|
|
|
|
again:
|
|
v, ok := as.m.Load(outputKey)
|
|
if !ok {
|
|
// The entry is missing in the map. Try creating it.
|
|
v = &totalStateValue{
|
|
lastValues: make(map[string]*lastValueState),
|
|
}
|
|
outputKey = strings.Clone(outputKey)
|
|
vNew, loaded := as.m.LoadOrStore(outputKey, v)
|
|
if loaded {
|
|
// Use the entry created by a concurrent goroutine.
|
|
v = vNew
|
|
}
|
|
}
|
|
sv := v.(*totalStateValue)
|
|
sv.mu.Lock()
|
|
deleted := sv.deleted
|
|
if !deleted {
|
|
lv, ok := sv.lastValues[inputKey]
|
|
if !ok {
|
|
lv = &lastValueState{}
|
|
inputKey = strings.Clone(inputKey)
|
|
sv.lastValues[inputKey] = lv
|
|
}
|
|
if ok || as.keepFirstSample {
|
|
if s.value >= lv.value {
|
|
sv.total += s.value - lv.value
|
|
} else {
|
|
// counter reset
|
|
sv.total += s.value
|
|
}
|
|
}
|
|
lv.value = s.value
|
|
lv.deleteDeadline = deleteDeadline
|
|
sv.deleteDeadline = deleteDeadline
|
|
}
|
|
sv.mu.Unlock()
|
|
if deleted {
|
|
// The entry has been deleted by the concurrent call to flushState
|
|
// Try obtaining and updating the entry again.
|
|
goto again
|
|
}
|
|
}
|
|
}
|
|
|
|
func (as *totalAggrState) removeOldEntries(currentTime uint64) {
|
|
m := &as.m
|
|
m.Range(func(k, v interface{}) bool {
|
|
sv := v.(*totalStateValue)
|
|
|
|
sv.mu.Lock()
|
|
deleted := currentTime > sv.deleteDeadline
|
|
if deleted {
|
|
// Mark the current entry as deleted
|
|
sv.deleted = deleted
|
|
} else {
|
|
// Delete outdated entries in sv.lastValues
|
|
m := sv.lastValues
|
|
for k1, v1 := range m {
|
|
if currentTime > v1.deleteDeadline {
|
|
delete(m, k1)
|
|
}
|
|
}
|
|
}
|
|
sv.mu.Unlock()
|
|
|
|
if deleted {
|
|
m.Delete(k)
|
|
}
|
|
return true
|
|
})
|
|
}
|
|
|
|
func (as *totalAggrState) flushState(ctx *flushCtx) {
|
|
currentTime := fasttime.UnixTimestamp()
|
|
currentTimeMsec := int64(currentTime) * 1000
|
|
|
|
as.removeOldEntries(currentTime)
|
|
|
|
m := &as.m
|
|
m.Range(func(k, v interface{}) bool {
|
|
sv := v.(*totalStateValue)
|
|
sv.mu.Lock()
|
|
total := sv.total
|
|
if as.resetTotalOnFlush {
|
|
sv.total = 0
|
|
} else if math.Abs(sv.total) >= (1 << 53) {
|
|
// It is time to reset the entry, since it starts losing float64 precision
|
|
sv.total = 0
|
|
}
|
|
deleted := sv.deleted
|
|
sv.mu.Unlock()
|
|
if !deleted {
|
|
key := k.(string)
|
|
ctx.appendSeries(key, as.suffix, currentTimeMsec, total)
|
|
}
|
|
return true
|
|
})
|
|
}
|