mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
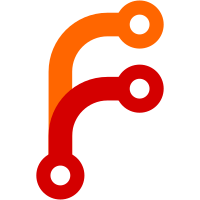
### Describe Your Changes This PR continues the implementation of integration tests (#7199). It adds the support for vm-single: - A vmsingle app wrapper has been added - Sample vmsingle tests that test the VM documentation related to querying data (#7435) - The tests use the go-cmp/{cmp,/cmpopts} packages, therefore they have been added to ./vendor - Minor refactoring: data objects have been moved to model.go Advice on porting things to cluster branch: - The build rule must include tests that start with TestVmsingle (similarly to how TestCluster tests are skipped in master branch) - The build rule must depend on `vmstorage vminsert vmselect` instead of `victoria-metrics` - The query_test.go can actually be implemented for cluster as well. To do this the tests need to be renamed to start with TestCluster and the tests must instantiace vm{storage,insert,select} instead of vmsingle. ### Checklist The following checks are **mandatory**: - [x] My change adheres [VictoriaMetrics contributing guidelines](https://docs.victoriametrics.com/contributing/). --------- Signed-off-by: Artem Fetishev <rtm@victoriametrics.com> Signed-off-by: hagen1778 <roman@victoriametrics.com> Co-authored-by: hagen1778 <roman@victoriametrics.com>
68 lines
2.1 KiB
Go
68 lines
2.1 KiB
Go
package tests
|
|
|
|
import (
|
|
"fmt"
|
|
"math/rand/v2"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/apptest"
|
|
)
|
|
|
|
func TestClusterMultilevelSelect(t *testing.T) {
|
|
tc := apptest.NewTestCase(t)
|
|
defer tc.Close()
|
|
|
|
// Set up the following multi-level cluster configuration:
|
|
//
|
|
// vmselect (L2) -> vmselect (L1) -> vmstorage <- vminsert
|
|
//
|
|
// vmisert writes data into vmstorage.
|
|
// vmselect (L2) reads that data via vmselect (L1).
|
|
|
|
cli := tc.Client()
|
|
|
|
vmstorage := apptest.MustStartVmstorage(t, "vmstorage", []string{
|
|
"-storageDataPath=" + tc.Dir() + "/vmstorage",
|
|
}, cli)
|
|
defer vmstorage.Stop()
|
|
vminsert := apptest.MustStartVminsert(t, "vminsert", []string{
|
|
"-storageNode=" + vmstorage.VminsertAddr(),
|
|
}, cli)
|
|
defer vminsert.Stop()
|
|
vmselectL1 := apptest.MustStartVmselect(t, "vmselect-level1", []string{
|
|
"-storageNode=" + vmstorage.VmselectAddr(),
|
|
}, cli)
|
|
defer vmselectL1.Stop()
|
|
vmselectL2 := apptest.MustStartVmselect(t, "vmselect-level2", []string{
|
|
"-storageNode=" + vmselectL1.ClusternativeListenAddr(),
|
|
}, cli)
|
|
defer vmselectL2.Stop()
|
|
|
|
// Insert 1000 unique time series.Wait for 2 seconds to let vmstorage
|
|
// flush pending items so they become searchable.
|
|
|
|
const numMetrics = 1000
|
|
records := make([]string, numMetrics)
|
|
for i := range numMetrics {
|
|
records[i] = fmt.Sprintf("metric_%d %d", i, rand.IntN(1000))
|
|
}
|
|
vminsert.PrometheusAPIV1ImportPrometheus(t, records, apptest.QueryOpts{Tenant: "0"})
|
|
time.Sleep(2 * time.Second)
|
|
|
|
// Retrieve all time series and verify that vmselect (L1) serves the complete
|
|
// set of time series.
|
|
|
|
seriesL1 := vmselectL1.PrometheusAPIV1Series(t, `{__name__=~".*"}`, apptest.QueryOpts{Tenant: "0"})
|
|
if got, want := len(seriesL1.Data), numMetrics; got != want {
|
|
t.Fatalf("unexpected level-1 series count: got %d, want %d", got, want)
|
|
}
|
|
|
|
// Retrieve all time series and verify that vmselect (L2) serves the complete
|
|
// set of time series.
|
|
|
|
seriesL2 := vmselectL2.PrometheusAPIV1Series(t, `{__name__=~".*"}`, apptest.QueryOpts{Tenant: "0"})
|
|
if got, want := len(seriesL2.Data), numMetrics; got != want {
|
|
t.Fatalf("unexpected level-2 series count: got %d, want %d", got, want)
|
|
}
|
|
}
|