mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
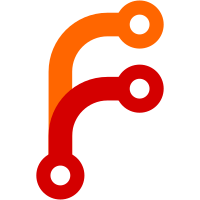
* vmalert: support rules backfilling (aka `replay`) vmalert can `replay` configured rules in the past and backfill results via remote write protocol. It supports MetricsQL/PromQL storage as data source, and can backfill data to remote write compatible storage. Supports recording and alerting rules `replay`. See more details in README. https://github.com/VictoriaMetrics/VictoriaMetrics/issues/836 * vmalert: review fixes * vmalert: readme fixes
66 lines
1.5 KiB
Go
66 lines
1.5 KiB
Go
package datasource
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
)
|
|
|
|
// Querier interface wraps Query and QueryRange methods
|
|
type Querier interface {
|
|
Query(ctx context.Context, query string) ([]Metric, error)
|
|
QueryRange(ctx context.Context, query string, from, to time.Time) ([]Metric, error)
|
|
}
|
|
|
|
// QuerierBuilder builds Querier with given params.
|
|
type QuerierBuilder interface {
|
|
BuildWithParams(params QuerierParams) Querier
|
|
}
|
|
|
|
// QuerierParams params for Querier.
|
|
type QuerierParams struct {
|
|
DataSourceType *Type
|
|
EvaluationInterval time.Duration
|
|
// see https://docs.victoriametrics.com/#prometheus-querying-api-enhancements
|
|
ExtraLabels map[string]string
|
|
}
|
|
|
|
// Metric is the basic entity which should be return by datasource
|
|
type Metric struct {
|
|
Labels []Label
|
|
Timestamps []int64
|
|
Values []float64
|
|
}
|
|
|
|
// SetLabel adds or updates existing one label
|
|
// by the given key and label
|
|
func (m *Metric) SetLabel(key, value string) {
|
|
for i, l := range m.Labels {
|
|
if l.Name == key {
|
|
m.Labels[i].Value = value
|
|
return
|
|
}
|
|
}
|
|
m.AddLabel(key, value)
|
|
}
|
|
|
|
// AddLabel appends the given label to the label set
|
|
func (m *Metric) AddLabel(key, value string) {
|
|
m.Labels = append(m.Labels, Label{Name: key, Value: value})
|
|
}
|
|
|
|
// Label returns the given label value.
|
|
// If label is missing empty string will be returned
|
|
func (m *Metric) Label(key string) string {
|
|
for _, l := range m.Labels {
|
|
if l.Name == key {
|
|
return l.Value
|
|
}
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// Label represents metric's label
|
|
type Label struct {
|
|
Name string
|
|
Value string
|
|
}
|