mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
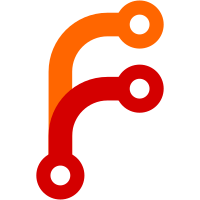
Prevsiously every aggregation output was using its own timestamp for the output aggregated samples in a single aggregation interval. This could result in unexpected inconsitent timesetamps for the output aggregated samples. This commit consistently uses the same timestamp across all the output aggregated samples. This commit makes sure that the duration between subsequent timestamps strictly equals the configured aggregation interval. Thanks to @AndrewChubatiuk for the original idea at https://github.com/VictoriaMetrics/VictoriaMetrics/pull/6314 This commit should help https://github.com/VictoriaMetrics/VictoriaMetrics/issues/4580
81 lines
1.8 KiB
Go
81 lines
1.8 KiB
Go
package streamaggr
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/bytesutil"
|
|
)
|
|
|
|
// sumSamplesAggrState calculates output=sum_samples, e.g. the sum over input samples.
|
|
type sumSamplesAggrState struct {
|
|
m sync.Map
|
|
}
|
|
|
|
type sumSamplesStateValue struct {
|
|
mu sync.Mutex
|
|
sum float64
|
|
deleted bool
|
|
}
|
|
|
|
func newSumSamplesAggrState() *sumSamplesAggrState {
|
|
return &sumSamplesAggrState{}
|
|
}
|
|
|
|
func (as *sumSamplesAggrState) pushSamples(samples []pushSample) {
|
|
for i := range samples {
|
|
s := &samples[i]
|
|
outputKey := getOutputKey(s.key)
|
|
|
|
again:
|
|
v, ok := as.m.Load(outputKey)
|
|
if !ok {
|
|
// The entry is missing in the map. Try creating it.
|
|
v = &sumSamplesStateValue{
|
|
sum: s.value,
|
|
}
|
|
outputKey = bytesutil.InternString(outputKey)
|
|
vNew, loaded := as.m.LoadOrStore(outputKey, v)
|
|
if !loaded {
|
|
// The new entry has been successfully created.
|
|
continue
|
|
}
|
|
// Use the entry created by a concurrent goroutine.
|
|
v = vNew
|
|
}
|
|
sv := v.(*sumSamplesStateValue)
|
|
sv.mu.Lock()
|
|
deleted := sv.deleted
|
|
if !deleted {
|
|
sv.sum += s.value
|
|
}
|
|
sv.mu.Unlock()
|
|
if deleted {
|
|
// The entry has been deleted by the concurrent call to flushState
|
|
// Try obtaining and updating the entry again.
|
|
goto again
|
|
}
|
|
}
|
|
}
|
|
|
|
func (as *sumSamplesAggrState) flushState(ctx *flushCtx, resetState bool) {
|
|
m := &as.m
|
|
m.Range(func(k, v any) bool {
|
|
if resetState {
|
|
// Atomically delete the entry from the map, so new entry is created for the next flush.
|
|
m.Delete(k)
|
|
}
|
|
|
|
sv := v.(*sumSamplesStateValue)
|
|
sv.mu.Lock()
|
|
sum := sv.sum
|
|
if resetState {
|
|
// Mark the entry as deleted, so it won't be updated anymore by concurrent pushSample() calls.
|
|
sv.deleted = true
|
|
}
|
|
sv.mu.Unlock()
|
|
|
|
key := k.(string)
|
|
ctx.appendSeries(key, "sum_samples", sum)
|
|
return true
|
|
})
|
|
}
|