mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
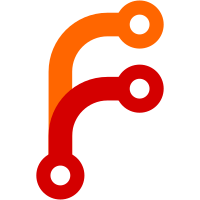
Previously filters wheren't passed to this call after the commit 0e09fdb8b0
Updates https://github.com/VictoriaMetrics/VictoriaMetrics/issues/1626
45 lines
960 B
Go
45 lines
960 B
Go
package ec2
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/awsapi"
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promscrape/discoveryutils"
|
|
)
|
|
|
|
type apiConfig struct {
|
|
awsConfig *awsapi.Config
|
|
filters []awsapi.Filter
|
|
port int
|
|
|
|
// A map from AZ name to AZ id.
|
|
azMap map[string]string
|
|
azMapLock sync.Mutex
|
|
}
|
|
|
|
var configMap = discoveryutils.NewConfigMap()
|
|
|
|
func getAPIConfig(sdc *SDConfig) (*apiConfig, error) {
|
|
v, err := configMap.Get(sdc, func() (interface{}, error) { return newAPIConfig(sdc) })
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return v.(*apiConfig), nil
|
|
}
|
|
|
|
func newAPIConfig(sdc *SDConfig) (*apiConfig, error) {
|
|
port := 80
|
|
if sdc.Port != nil {
|
|
port = *sdc.Port
|
|
}
|
|
awsCfg, err := awsapi.NewConfig(sdc.Region, sdc.RoleARN, sdc.AccessKey, sdc.SecretKey.String())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
cfg := &apiConfig{
|
|
awsConfig: awsCfg,
|
|
filters: sdc.Filters,
|
|
port: port,
|
|
}
|
|
return cfg, nil
|
|
}
|