mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
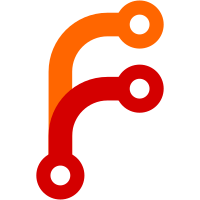
Previous commit201fd6de1e
removed trailing space trim from data read from file. But common practice is to remove such trailing space. And it leaded to the authorization errors for the major group of users. In first place, this change must help to mitigate an issue with kubernetes. When authorization information was read from Secret content. Changes to the operator was made to mitigate such problem at commit1cf64358c8
We could introduce later optional flag for VictoriaMetrics to disable trim space behavior. Related issues: https://github.com/VictoriaMetrics/VictoriaMetrics/issues/6986 https://github.com/VictoriaMetrics/VictoriaMetrics/issues/7089 https://github.com/VictoriaMetrics/VictoriaMetrics/issues/6947 --------- Signed-off-by: f41gh7 <nik@victoriametrics.com> Co-authored-by: Zhu Jiekun <jiekun@victoriametrics.com>
81 lines
2.4 KiB
Go
81 lines
2.4 KiB
Go
package flagutil
|
|
|
|
import (
|
|
"path/filepath"
|
|
"testing"
|
|
)
|
|
|
|
func TestPassword(t *testing.T) {
|
|
p := Password{
|
|
flagname: "foo",
|
|
}
|
|
|
|
// Verify that String returns "secret"
|
|
expectedSecret := "secret"
|
|
if s := p.String(); s != expectedSecret {
|
|
t.Fatalf("unexpected value returned from Password.String; got %q; want %q", s, expectedSecret)
|
|
}
|
|
|
|
// set regular password
|
|
expectedPassword := "top-secret-password"
|
|
if err := p.Set(expectedPassword); err != nil {
|
|
t.Fatalf("cannot set password: %s", err)
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
if s := p.Get(); s != expectedPassword {
|
|
t.Fatalf("unexpected password; got %q; want %q", s, expectedPassword)
|
|
}
|
|
if s := p.String(); s != expectedSecret {
|
|
t.Fatalf("unexpected value returned from Password.String; got %q; want %q", s, expectedSecret)
|
|
}
|
|
}
|
|
|
|
// read the password from file by relative path
|
|
localPassFile := "testdata/password.txt"
|
|
expectedPassword = "foo-bar-baz"
|
|
path := "file://" + localPassFile
|
|
if err := p.Set(path); err != nil {
|
|
t.Fatalf("cannot set password to file: %s", err)
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
if s := p.Get(); s != expectedPassword {
|
|
t.Fatalf("unexpected password; got %q; want %q", s, expectedPassword)
|
|
}
|
|
if s := p.String(); s != expectedSecret {
|
|
t.Fatalf("unexpected value returned from Password.String; got %q; want %q", s, expectedSecret)
|
|
}
|
|
}
|
|
|
|
// read the password from file by absolute path
|
|
var err error
|
|
localPassFile, err = filepath.Abs("testdata/password.txt")
|
|
if err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
expectedPassword = "foo-bar-baz"
|
|
path = "file://" + localPassFile
|
|
if err := p.Set(path); err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
if s := p.Get(); s != expectedPassword {
|
|
t.Fatalf("unexpected password; got %q; want %q", s, expectedPassword)
|
|
}
|
|
if s := p.String(); s != expectedSecret {
|
|
t.Fatalf("unexpected value returned from Password.String; got %q; want %q", s, expectedSecret)
|
|
}
|
|
}
|
|
|
|
// try reading the password from non-existing url
|
|
if err := p.Set("http://127.0.0.1:56283/aaa/bb?cc=dd"); err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
if s := p.Get(); len(s) != 64 {
|
|
t.Fatalf("unexpected password obtained: %q; must be random 64-byte password", s)
|
|
}
|
|
if s := p.String(); s != expectedSecret {
|
|
t.Fatalf("unexpected value returned from Password.String; got %q; want %q", s, expectedSecret)
|
|
}
|
|
}
|
|
}
|