mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
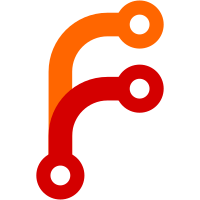
- Return meta-labels for the discovered targets via promutils.Labels instead of map[string]string. This improves the speed of generating meta-labels for discovered targets by up to 5x. - Remove memory allocations in hot paths during ScrapeWork generation. The ScrapeWork contains scrape settings for a single discovered target. This improves the service discovery speed by up to 2x.
51 lines
1.1 KiB
Go
51 lines
1.1 KiB
Go
package http
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/promutils"
|
|
)
|
|
|
|
func Test_parseAPIResponse(t *testing.T) {
|
|
type args struct {
|
|
data []byte
|
|
path string
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want []httpGroupTarget
|
|
wantErr bool
|
|
}{
|
|
|
|
{
|
|
name: "parse ok",
|
|
args: args{
|
|
path: "/ok",
|
|
data: []byte(`[
|
|
{"targets": ["http://target-1:9100","http://target-2:9150"],
|
|
"labels": {"label-1":"value-1"} }
|
|
]`),
|
|
},
|
|
want: []httpGroupTarget{
|
|
{
|
|
Labels: promutils.NewLabelsFromMap(map[string]string{"label-1": "value-1"}),
|
|
Targets: []string{"http://target-1:9100", "http://target-2:9150"},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got, err := parseAPIResponse(tt.args.data, tt.args.path)
|
|
if (err != nil) != tt.wantErr {
|
|
t.Errorf("parseAPIResponse() error = %v, wantErr %v", err, tt.wantErr)
|
|
return
|
|
}
|
|
if !reflect.DeepEqual(got, tt.want) {
|
|
t.Errorf("parseAPIResponse() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|