mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
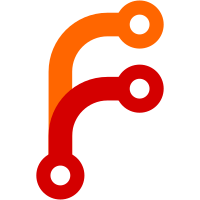
The %q formatter may result in incorrectly formatted JSON string if the original string
contains special chars such as \x1b . They must be encoded as \u001b , otherwise the resulting JSON string
cannot be parsed by JSON parsers.
This is a follow-up for c0caa69939
See https://github.com/VictoriaMetrics/victorialogs-datasource/issues/24
28 lines
831 B
Go
28 lines
831 B
Go
package firehose
|
|
|
|
import (
|
|
"fmt"
|
|
"net/http"
|
|
"time"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/stringsutil"
|
|
)
|
|
|
|
// WriteSuccessResponse writes success response for AWS Firehose request.
|
|
//
|
|
// See https://docs.aws.amazon.com/firehose/latest/dev/httpdeliveryrequestresponse.html#responseformat
|
|
func WriteSuccessResponse(w http.ResponseWriter, r *http.Request) {
|
|
requestID := r.Header.Get("X-Amz-Firehose-Request-Id")
|
|
if requestID == "" {
|
|
// This isn't a AWS firehose request - just return an empty response in this case.
|
|
w.WriteHeader(http.StatusOK)
|
|
return
|
|
}
|
|
|
|
body := fmt.Sprintf(`{"requestId":%s,"timestamp":%d}`, stringsutil.JSONString(requestID), time.Now().UnixMilli())
|
|
|
|
h := w.Header()
|
|
h.Set("Content-Type", "application/json")
|
|
h.Set("Content-Length", fmt.Sprintf("%d", len(body)))
|
|
w.Write([]byte(body))
|
|
}
|