mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
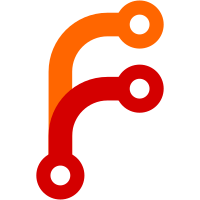
* lib/promscrape: support prometheus-like duration in scrape configs The change allows to specify duration values like `1d`, `1w` for fields `scrape_interval`, `scrape_timeout`, etc. https://github.com/VictoriaMetrics/VictoriaMetrics/issues/817#issuecomment-1033384766 Signed-off-by: hagen1778 <roman@victoriametrics.com> * lib/blockcache: make linter happy Signed-off-by: hagen1778 <roman@victoriametrics.com> * lib/promscrape: support prometheus-like duration in scrape configs * add support for extra fields `scrape_align_interval` and `scrape_offset`; * support Prometheus duration parsing for `__scrape_interval__` and `__scrape_duration__` labels; Signed-off-by: hagen1778 <roman@victoriametrics.com> * wip * wip * docs/CHANGELOG.md: document the feature Co-authored-by: Aliaksandr Valialkin <valyala@victoriametrics.com>
42 lines
1.1 KiB
Go
42 lines
1.1 KiB
Go
package promutils
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestDuration(t *testing.T) {
|
|
if _, err := ParseDuration("foobar"); err == nil {
|
|
t.Fatalf("expecting error for invalid duration")
|
|
}
|
|
dNative, err := ParseDuration("1w")
|
|
if err != nil {
|
|
t.Fatalf("unexpected error: %s", err)
|
|
}
|
|
if dNative != 7*24*time.Hour {
|
|
t.Fatalf("unexpected duration; got %s; want %s", dNative, 7*24*time.Hour)
|
|
}
|
|
d := NewDuration(dNative)
|
|
if d.Duration() != dNative {
|
|
t.Fatalf("unexpected duration; got %s; want %s", d.Duration(), dNative)
|
|
}
|
|
v, err := d.MarshalYAML()
|
|
if err != nil {
|
|
t.Fatalf("unexpected error in MarshalYAML(): %s", err)
|
|
}
|
|
sExpected := "168h0m0s"
|
|
if s := v.(string); s != sExpected {
|
|
t.Fatalf("unexpected value from MarshalYAML(); got %q; want %q", s, sExpected)
|
|
}
|
|
if err := d.UnmarshalYAML(func(v interface{}) error {
|
|
sp := v.(*string)
|
|
s := "1w3d5h"
|
|
*sp = s
|
|
return nil
|
|
}); err != nil {
|
|
t.Fatalf("unexpected error in UnmarshalYAML(): %s", err)
|
|
}
|
|
if dNative := d.Duration(); dNative != (10*24+5)*time.Hour {
|
|
t.Fatalf("unexpected value; got %s; want %s", dNative, (10*24+5)*time.Hour)
|
|
}
|
|
}
|