mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-21 14:44:00 +00:00
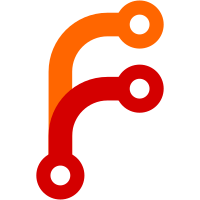
Sort labels explicitly after calling the ParsedConfigs.Apply() when needed. This reduces CPU usage when performing metric-level relabeling, where labels' sorting isn't needed.
54 lines
1,009 B
Go
54 lines
1,009 B
Go
package promrelabel
|
|
|
|
import (
|
|
"sort"
|
|
"sync"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/prompbmarshal"
|
|
)
|
|
|
|
// SortLabels sorts labels.
|
|
func SortLabels(labels []prompbmarshal.Label) {
|
|
if len(labels) < 2 {
|
|
return
|
|
}
|
|
ls := labelsSorterPool.Get().(*labelsSorter)
|
|
*ls = labels
|
|
if !sort.IsSorted(ls) {
|
|
sort.Sort(ls)
|
|
}
|
|
*ls = nil
|
|
labelsSorterPool.Put(ls)
|
|
}
|
|
|
|
// SortLabelsStable sorts labels using stable sort.
|
|
func SortLabelsStable(labels []prompbmarshal.Label) {
|
|
if len(labels) < 2 {
|
|
return
|
|
}
|
|
ls := labelsSorterPool.Get().(*labelsSorter)
|
|
*ls = labels
|
|
if !sort.IsSorted(ls) {
|
|
sort.Stable(ls)
|
|
}
|
|
*ls = nil
|
|
labelsSorterPool.Put(ls)
|
|
}
|
|
|
|
var labelsSorterPool = &sync.Pool{
|
|
New: func() interface{} {
|
|
return &labelsSorter{}
|
|
},
|
|
}
|
|
|
|
type labelsSorter []prompbmarshal.Label
|
|
|
|
func (ls *labelsSorter) Len() int { return len(*ls) }
|
|
func (ls *labelsSorter) Swap(i, j int) {
|
|
a := *ls
|
|
a[i], a[j] = a[j], a[i]
|
|
}
|
|
func (ls *labelsSorter) Less(i, j int) bool {
|
|
a := *ls
|
|
return a[i].Name < a[j].Name
|
|
}
|