mirror of
https://github.com/librespot-org/librespot.git
synced 2024-12-18 17:11:53 +00:00
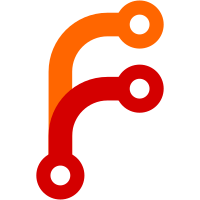
- Use variables directly in format strings. As reported by clippy, variables can be used directly in the `format!` string. - Use rewind() instead of seeking to 0. - Remove superfluous & and ref. Signed-off-by: Petr Tesarik <petr@tesarici.cz>
37 lines
1.1 KiB
Rust
37 lines
1.1 KiB
Rust
use std::fmt::Write;
|
|
|
|
use crate::MetadataError;
|
|
|
|
use librespot_core::{Error, Session};
|
|
|
|
pub type RequestResult = Result<bytes::Bytes, Error>;
|
|
|
|
#[async_trait]
|
|
pub trait MercuryRequest {
|
|
async fn request(session: &Session, uri: &str) -> RequestResult {
|
|
let mut metrics_uri = uri.to_owned();
|
|
|
|
let separator = match metrics_uri.find('?') {
|
|
Some(_) => "&",
|
|
None => "?",
|
|
};
|
|
let _ = write!(metrics_uri, "{separator}country={}", session.country());
|
|
|
|
if let Some(product) = session.get_user_attribute("type") {
|
|
let _ = write!(metrics_uri, "&product={product}");
|
|
}
|
|
|
|
trace!("Requesting {}", metrics_uri);
|
|
|
|
let request = session.mercury().get(metrics_uri)?;
|
|
let response = request.await?;
|
|
match response.payload.first() {
|
|
Some(data) => {
|
|
let data = data.to_vec().into();
|
|
trace!("Received metadata: {data:?}");
|
|
Ok(data)
|
|
}
|
|
None => Err(Error::unavailable(MetadataError::Empty)),
|
|
}
|
|
}
|
|
}
|