mirror of
https://github.com/librespot-org/librespot.git
synced 2024-12-18 17:11:53 +00:00
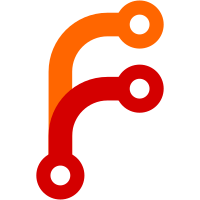
This was a huge effort by photovoltex@gmail.com with help from the community. Over 140 commits were squashed. Below, their commit messages are kept unchanged. --- * dealer wrapper for ease of use * improve sending protobuf requests * replace connect config with connect_state config * start integrating dealer into spirc * payload handling, gzip support * put connect state consistent * formatting * request payload handling, gzip support * expose dealer::protocol, move request in own file * integrate handle of connect-state commands * spirc: remove ident field * transfer playing state better * spirc: remove remote_update stream * spirc: replace command sender with connect state update * spirc: remove device state and remaining unused methods * spirc: remove mercury sender * add repeat track state * ConnectState: add methods to replace state in spirc * spirc: move context into connect_state, update load and next * spirc: remove state, adjust remaining methods * spirc: handle more dealer request commands * revert rustfmt.toml * spirc: impl shuffle - impl shuffle again - extracted fill up of next tracks in own method - moved queue revision update into next track fill up - removed unused method `set_playing_track_index` - added option to specify index when resetting the playback context - reshuffle after repeat context * spirc: handle device became inactive * dealer: adjust payload handling * spirc: better set volume handling * dealer: box PlayCommand (clippy warning) * dealer: always respect queued tracks * spirc: update duration of track * ConnectState: update more restrictions * cleanup * spirc: handle queue requests * spirc: skip next with track * proto: exclude spirc.proto - move "deserialize_with" functions into own file - replace TrackRef with ProvidedTrack * spirc: stabilize transfer/context handling * core: cleanup some remains * connect: improvements to code structure and performance - use VecDeque for next and prev tracks * connect: delayed volume update * connect: move context resolve into own function * connect: load context asynchronous * connect: handle reconnect - might currently steal the active devices playback * connect: some fixes and adjustments - fix wrong offset when transferring playback - fix missing displayed context in web-player - remove access_token from log - send correct state reason when updating volume - queue track correctly - fix wrong assumption for skip_to * connect: replace error case with option * connect: use own context state * connect: more stabilising - handle SkipTo having no Index - handle no transferred restrictions - handle no transferred index - update state before shutdown, for smoother reacquiring * connect: working autoplay * connect: handle repeat context/track * connect: some quick fixes - found self-named uid in collection after reconnecting * connect: handle add_to_queue via set_queue * fix clippy warnings * fix check errors, fix/update example * fix 1.75 specific error * connect: position update improvements * connect: handle unavailable * connect: fix incorrect status handling for desktop and mobile * core: fix dealer reconnect - actually acquire new token - use login5 token retrieval * connect: split state into multiple files * connect: encapsulate provider logic * connect: remove public access to next and prev tracks * connect: remove public access to player * connect: move state only commands into own file * connect: improve logging * connect: handle transferred queue again * connect: fix all-features specific error * connect: extract transfer handling into own file * connect: remove old context model * connect: handle more transfer cases correctly * connect: do auth_token pre-acquiring earlier * connect: handle play with skip_to by uid * connect: simplified cluster update log * core/connect: add remaining set value commands * connect: position update workaround/fix * connect: some queue cleanups * connect: add uid to queue * connect: duration as volume delay const * connect: some adjustments and todo cleanups - send volume update before general update - simplify queue revision to use the track uri - argument why copying the prev/next tracks is fine * connect: handle shuffle from set_options * connect: handle context update * connect: move other structs into model.rs * connect: reduce SpircCommand visibility * connect: fix visibility of model * connect: fix: shuffle on startup isn't applied * connect: prevent loading a context with no tracks * connect: use the first page of a context * connect: improve context resolving - support multiple pages - support page_url of context - handle single track * connect: prevent integer underflow * connect: rename method for better clarity * connect: handle mutate and update messages * connect: fix 1.75 problems * connect: fill, instead of replace next page * connect: reduce context update to single method * connect: remove unused SpircError, handle local files * connect: reduce nesting, adjust initial transfer handling * connect: don't update volume initially * core: disable trace logging of handled mercury responses * core/connect: prevent takeover from other clients, handle session-update * connect: add queue-uid for set_queue command * connect: adjust fields for PlayCommand * connect: preserve context position after update_context * connect: unify metadata modification - only handle `is_queued` `true` items for queue * connect: polish request command handling - reply to all request endpoints - adjust some naming - add some docs * connect: add uid to tracks without * connect: simpler update of current index * core/connect: update log msg, fix wrong behavior - handle became inactive separately - remove duplicate stop - adjust docs for websocket request * core: add option to request without metrics and salt * core/context: adjust context requests and update - search should now return the expected context - removed workaround for single track playback - move local playback check into update_context - check track uri for invalid characters - early return with `?` * connect: handle possible search context uri * connect: remove logout support - handle logout command - disable support for logout - add todos for logout * connect: adjust detailed tracks/context handling - always allow next - handle no prev track available - separate active and fill up context * connect: adjust context resolve handling, again * connect: add autoplay metadata to tracks - transfer into autoplay again * core/connect: cleanup session after spirc stops * update CHANGELOG.md * playback: fix clippy warnings * connect: adjust metadata - unify naming - move more metadata infos into metadata.rs * connect: add delimiter between context and autoplay playback * connect: stop and resume correctly * connect: adjust context resolving - improved certain logging parts - preload autoplay when autoplay attribute mutates - fix transfer context uri - fix typo - handle empty strings for resolve uri - fix unexpected stop of playback * connect: ignore failure during stop * connect: revert resolve_uri changes * connect: correct context reset * connect: reduce boiler code * connect: fix some incorrect states - uid getting replaced by empty value - shuffle/repeat clearing autoplay context - fill_up updating and using incorrect index * core: adjust incorrect separator * connect: move `add_to_queue` and `mark_unavailable` into tracks.rs * connect: refactor - directly modify PutStateRequest - replace `next_tracks`, `prev_tracks`, `player` and `device` with `request` - provide helper methods for the removed fields * connect: adjust handling of context metadata/restrictions * connect: fix incorrect context states * connect: become inactive when no cluster is reported * update CHANGELOG.md * core/playback: preemptively fix clippy warnings * connect: minor adjustment to session changed * connect: change return type changing active context * connect: handle unavailable contexts * connect: fix previous restrictions blocking load with shuffle * connect: update comments and logging * core/connect: reduce some more duplicate code * more docs around the dealer
69 lines
2.4 KiB
Rust
69 lines
2.4 KiB
Rust
use std::{
|
|
env, fs,
|
|
ops::Deref,
|
|
path::{Path, PathBuf},
|
|
};
|
|
|
|
fn out_dir() -> PathBuf {
|
|
Path::new(&env::var("OUT_DIR").expect("env")).to_path_buf()
|
|
}
|
|
|
|
fn cleanup() {
|
|
let _ = fs::remove_dir_all(out_dir());
|
|
}
|
|
|
|
fn compile() {
|
|
let proto_dir = Path::new(&env::var("CARGO_MANIFEST_DIR").expect("env")).join("proto");
|
|
|
|
let files = &[
|
|
proto_dir.join("connect.proto"),
|
|
proto_dir.join("connectivity.proto"),
|
|
proto_dir.join("devices.proto"),
|
|
proto_dir.join("entity_extension_data.proto"),
|
|
proto_dir.join("extended_metadata.proto"),
|
|
proto_dir.join("extension_kind.proto"),
|
|
proto_dir.join("metadata.proto"),
|
|
proto_dir.join("player.proto"),
|
|
proto_dir.join("playlist_annotate3.proto"),
|
|
proto_dir.join("playlist_permission.proto"),
|
|
proto_dir.join("playlist4_external.proto"),
|
|
proto_dir.join("spotify/clienttoken/v0/clienttoken_http.proto"),
|
|
proto_dir.join("spotify/login5/v3/challenges/code.proto"),
|
|
proto_dir.join("spotify/login5/v3/challenges/hashcash.proto"),
|
|
proto_dir.join("spotify/login5/v3/client_info.proto"),
|
|
proto_dir.join("spotify/login5/v3/credentials/credentials.proto"),
|
|
proto_dir.join("spotify/login5/v3/identifiers/identifiers.proto"),
|
|
proto_dir.join("spotify/login5/v3/login5.proto"),
|
|
proto_dir.join("spotify/login5/v3/user_info.proto"),
|
|
proto_dir.join("storage-resolve.proto"),
|
|
proto_dir.join("user_attributes.proto"),
|
|
proto_dir.join("autoplay_context_request.proto"),
|
|
proto_dir.join("social_connect_v2.proto"),
|
|
// TODO: remove these legacy protobufs when we are on the new API completely
|
|
proto_dir.join("authentication.proto"),
|
|
proto_dir.join("canvaz.proto"),
|
|
proto_dir.join("canvaz-meta.proto"),
|
|
proto_dir.join("explicit_content_pubsub.proto"),
|
|
proto_dir.join("keyexchange.proto"),
|
|
proto_dir.join("mercury.proto"),
|
|
proto_dir.join("pubsub.proto"),
|
|
];
|
|
|
|
let slices = files.iter().map(Deref::deref).collect::<Vec<_>>();
|
|
|
|
let out_dir = out_dir();
|
|
fs::create_dir(&out_dir).expect("create_dir");
|
|
|
|
protobuf_codegen::Codegen::new()
|
|
.pure()
|
|
.out_dir(&out_dir)
|
|
.inputs(&slices)
|
|
.include(&proto_dir)
|
|
.run()
|
|
.expect("Codegen failed.");
|
|
}
|
|
|
|
fn main() {
|
|
cleanup();
|
|
compile();
|
|
}
|