mirror of
https://github.com/librespot-org/librespot.git
synced 2024-12-28 17:21:52 +00:00
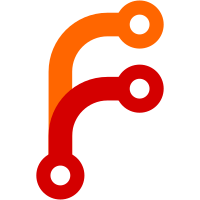
* create Volume struct for use with Cache * add "volume" file to Cache * load cached volume on start, intial overrides cached overrides default * amend volume_to_mixer function to cache the volume on every change * pass cache to Spirc and SpircTask so volume_to_mixer has access * rustfmt changes * revert volume_to_mixer function and Spirc/SpircTask cache variable * Volume implements Copy, pass by value instead of reference * clamp volume to 100 if cached value exceeds limit * convert Volume to u16 internally, use float and round to convert hex->dec * convert initial_volume and ConnectConfig.volume to u16 as well * add cache_volume function to SpircTask * remove conversion to/from percentage on cached volume * consolidate device.set_volume, mixer.set_volume, and caching * streamline intial volume logic
31 lines
849 B
Rust
31 lines
849 B
Rust
use std::fs::File;
|
|
use std::io::{Read, Write};
|
|
use std::path::Path;
|
|
|
|
#[derive(Clone, Copy, Debug)]
|
|
pub struct Volume {
|
|
pub volume: u16,
|
|
}
|
|
|
|
impl Volume {
|
|
// read volume from file
|
|
fn from_reader<R: Read>(mut reader: R) -> u16 {
|
|
let mut contents = String::new();
|
|
reader.read_to_string(&mut contents).unwrap();
|
|
contents.trim().parse::<u16>().unwrap()
|
|
}
|
|
|
|
pub(crate) fn from_file<P: AsRef<Path>>(path: P) -> Option<u16> {
|
|
File::open(path).ok().map(Volume::from_reader)
|
|
}
|
|
|
|
// write volume to file
|
|
fn save_to_writer<W: Write>(&self, writer: &mut W) {
|
|
writer.write_all(self.volume.to_string().as_bytes()).unwrap();
|
|
}
|
|
|
|
pub(crate) fn save_to_file<P: AsRef<Path>>(&self, path: P) {
|
|
let mut file = File::create(path).unwrap();
|
|
self.save_to_writer(&mut file)
|
|
}
|
|
}
|