mirror of
https://github.com/librespot-org/librespot.git
synced 2024-11-08 16:45:43 +00:00
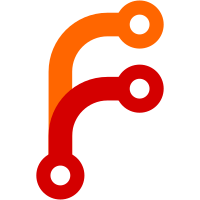
Special thanks to @eladyn for all of their help and suggestions. * Add all player events to `player_event_handler.rs` * Move event handler code to `player_event_handler.rs` * Add session events * Clean up and de-noise events and event firing * Added metadata support via a TrackChanged event * Add `event_handler_example.py` * Handle invalid track start positions by just starting the track from the beginning * Add repeat support to `spirc.rs` * Add `disconnect`, `set_position_ms` and `set_volume` to `spirc.rs` * Set `PlayStatus` to the correct value when Player is loading to avoid blanking out the controls when `self.play_status` is `LoadingPlay` or `LoadingPause` in `spirc.rs` * Handle attempts to play local files better by basically ignoring attempts to load them in `handle_remote_update` in `spirc.rs` * Add an event worker thread that runs async to the main thread(s) but sync to itself to prevent potential data races for event consumers. * Get rid of (probably harmless) `.unwrap()` in `main.rs` * Ensure that events are emited in a logical order and at logical times * Handle invalid and disappearing devices better * Ignore SpircCommands unless we're active with the exception of ShutDown
50 lines
1.3 KiB
Rust
50 lines
1.3 KiB
Rust
use std::{env, process::exit};
|
|
|
|
use librespot::{
|
|
core::{
|
|
authentication::Credentials, config::SessionConfig, session::Session, spotify_id::SpotifyId,
|
|
},
|
|
playback::{
|
|
audio_backend,
|
|
config::{AudioFormat, PlayerConfig},
|
|
mixer::NoOpVolume,
|
|
player::Player,
|
|
},
|
|
};
|
|
|
|
#[tokio::main]
|
|
async fn main() {
|
|
let session_config = SessionConfig::default();
|
|
let player_config = PlayerConfig::default();
|
|
let audio_format = AudioFormat::default();
|
|
|
|
let args: Vec<_> = env::args().collect();
|
|
if args.len() != 4 {
|
|
eprintln!("Usage: {} USERNAME PASSWORD TRACK", args[0]);
|
|
return;
|
|
}
|
|
let credentials = Credentials::with_password(&args[1], &args[2]);
|
|
|
|
let track = SpotifyId::from_base62(&args[3]).unwrap();
|
|
|
|
let backend = audio_backend::find(None).unwrap();
|
|
|
|
println!("Connecting...");
|
|
let session = Session::new(session_config, None);
|
|
if let Err(e) = session.connect(credentials, false).await {
|
|
println!("Error connecting: {}", e);
|
|
exit(1);
|
|
}
|
|
|
|
let mut player = Player::new(player_config, session, Box::new(NoOpVolume), move || {
|
|
backend(None, audio_format)
|
|
});
|
|
|
|
player.load(track, true, 0);
|
|
|
|
println!("Playing...");
|
|
|
|
player.await_end_of_track().await;
|
|
|
|
println!("Done");
|
|
}
|