mirror of
https://github.com/librespot-org/librespot.git
synced 2024-12-18 17:11:53 +00:00
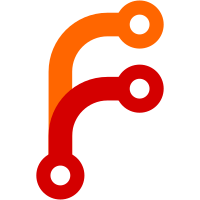
- `from_repeated_message` -> `impl_from_repeated` - `from_repeated_enum` -> `impl_from_repeated_copy` since the enum references were just simply deref copied - `try_from_repeated_message` -> `impl_try_from_repeated` - Simplified the implementation of `from_repeated_enum`
40 lines
1,018 B
Rust
40 lines
1,018 B
Rust
use std::{
|
|
convert::{TryFrom, TryInto},
|
|
fmt::Debug,
|
|
ops::{Deref, DerefMut},
|
|
};
|
|
|
|
use crate::{
|
|
restriction::Restrictions,
|
|
util::{impl_deref_wrapped, impl_try_from_repeated},
|
|
};
|
|
|
|
use librespot_core::date::Date;
|
|
|
|
use librespot_protocol as protocol;
|
|
use protocol::metadata::SalePeriod as SalePeriodMessage;
|
|
|
|
#[derive(Debug, Clone)]
|
|
pub struct SalePeriod {
|
|
pub restrictions: Restrictions,
|
|
pub start: Date,
|
|
pub end: Date,
|
|
}
|
|
|
|
#[derive(Debug, Clone, Default)]
|
|
pub struct SalePeriods(pub Vec<SalePeriod>);
|
|
|
|
impl_deref_wrapped!(SalePeriods, Vec<SalePeriod>);
|
|
|
|
impl TryFrom<&SalePeriodMessage> for SalePeriod {
|
|
type Error = librespot_core::Error;
|
|
fn try_from(sale_period: &SalePeriodMessage) -> Result<Self, Self::Error> {
|
|
Ok(Self {
|
|
restrictions: sale_period.get_restriction().into(),
|
|
start: sale_period.get_start().try_into()?,
|
|
end: sale_period.get_end().try_into()?,
|
|
})
|
|
}
|
|
}
|
|
|
|
impl_try_from_repeated!(SalePeriodMessage, SalePeriods);
|